MS CRM - Custom Workflow:
Below example shows how custom workflow is worked in MS CRM,
On Creation of Opportunity - Phone Call Activity has to be created and need to scheduled for next day, to do followups.
If particular opportunity created during weekdays it would be done next day, its fine. But opportunity creates on Friday or Saturday it will fall on weekends. In order to avoid this conflict-ion I am using custom workflows.
******************************************************************************
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Activities;
using Microsoft.Xrm.Sdk;
using Microsoft.Xrm.Sdk.Workflow;
using Microsoft.Crm.Sdk.Messages;
using Microsoft.Xrm.Sdk.Messages;
namespace PET.TEST.WF.DUEDATEWF
{
public sealed class UpdateDueDate : CodeActivity
{
protected override void Execute(CodeActivityContext context)
{
IWorkflowContext wfContext = context.GetExtension<IWorkflowContext>();
IOrganizationServiceFactory serviceFactory = context.GetExtension<IOrganizationServiceFactory>();
IOrganizationService service = serviceFactory.CreateOrganizationService(wfContext.UserId);
if (wfContext.MessageName.ToLower() == "create")
{
Entity opp = (Entity)wfContext.InputParameters["Target"];
Entity phoneCallActivity = new Entity("phonecall");
//DateTime dueDate = phaneCallActivity.GetAttributeValue<DateTime>("scheduledend");
DateTime currentDate = DateTime.Now;
DayOfWeek currentDay = currentDate.DayOfWeek;
string day = Convert.ToString(currentDay);
if (day.ToLower() == "friday" || day.ToLower() == "saturday")
{
if (day.ToLower() == "friday")
{
phoneCallActivity["scheduledend"] = currentDate.AddDays(3);
}
else if (day.ToLower() == "saturday")
{
phoneCallActivity["scheduledend"] = currentDate.AddDays(2);
}
}
else
{
phoneCallActivity["scheduledend"] = currentDate.AddDays(1);
}
EntityReference regardingobjectId = new EntityReference("opportunity", opp.Id);
phoneCallActivity["regardingobjectid"] = regardingobjectId;
phoneCallActivity["subject"] = "Test";
service.Create(phoneCallActivity);
}
}
}
}
-------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------
and trigger the workflow
Step:5 After click ok button. If the error occurs
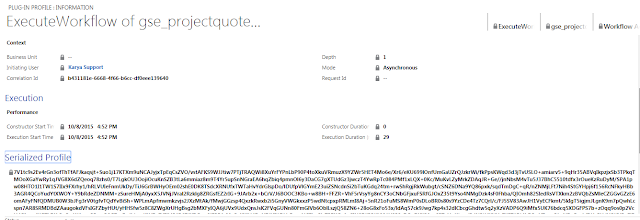
Step:8 Once you triggered the workflow. Go to "plugin profiles" and open relevant plugin record.
Step:9 Click on the “Serialized Profile” tab and copy the value specified in the “Serialized Profile”
.
Step 10. Create a new text file and copy the “Serialized Profile” value into it and then save the file.
Step 11. Go back to the Plugin Registration Tool, select the profile created earlier and click on the Debug button.
Step 12 In the opened Debug window, select the newly created text file in the Profile Location field and the Custom Workflow Activity
assembly in the Workflow Activity field.
Step 13 Open your Custom Workflow Activity project using Visual Studio.
Step 14 Put the break point in your code and attach the debugger to the Plugin Registration Tool process
and click the Start Execution button in the Plugin Registration Tool debug window.
The below steps are how to trigger the "OnDemand" workflow in the CRM
Step:1 Open any entity click "Run workflow".
Below example shows how custom workflow is worked in MS CRM,
On Creation of Opportunity - Phone Call Activity has to be created and need to scheduled for next day, to do followups.
If particular opportunity created during weekdays it would be done next day, its fine. But opportunity creates on Friday or Saturday it will fall on weekends. In order to avoid this conflict-ion I am using custom workflows.
- Start Visual Studio and select New Project->Workflow->Activity Library
- Delete “Activity1.xaml” file.
- Right Click on project and select Add New->add a class and name it “UpdateDueDate.cs”
- Right Click on project -> select properties and make sure Target Framework is “.Net Framework 4” under Application tab.
- Sign your assembly.
- Right Click on project and select Add Reference to add required assemblies to our project.
******************************************************************************
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Activities;
using Microsoft.Xrm.Sdk;
using Microsoft.Xrm.Sdk.Workflow;
using Microsoft.Crm.Sdk.Messages;
using Microsoft.Xrm.Sdk.Messages;
namespace PET.TEST.WF.DUEDATEWF
{
public sealed class UpdateDueDate : CodeActivity
{
protected override void Execute(CodeActivityContext context)
{
IWorkflowContext wfContext = context.GetExtension<IWorkflowContext>();
IOrganizationServiceFactory serviceFactory = context.GetExtension<IOrganizationServiceFactory>();
IOrganizationService service = serviceFactory.CreateOrganizationService(wfContext.UserId);
if (wfContext.MessageName.ToLower() == "create")
{
Entity opp = (Entity)wfContext.InputParameters["Target"];
Entity phoneCallActivity = new Entity("phonecall");
//DateTime dueDate = phaneCallActivity.GetAttributeValue<DateTime>("scheduledend");
DateTime currentDate = DateTime.Now;
DayOfWeek currentDay = currentDate.DayOfWeek;
string day = Convert.ToString(currentDay);
if (day.ToLower() == "friday" || day.ToLower() == "saturday")
{
if (day.ToLower() == "friday")
{
phoneCallActivity["scheduledend"] = currentDate.AddDays(3);
}
else if (day.ToLower() == "saturday")
{
phoneCallActivity["scheduledend"] = currentDate.AddDays(2);
}
}
else
{
phoneCallActivity["scheduledend"] = currentDate.AddDays(1);
}
EntityReference regardingobjectId = new EntityReference("opportunity", opp.Id);
phoneCallActivity["regardingobjectid"] = regardingobjectId;
phoneCallActivity["subject"] = "Test";
service.Create(phoneCallActivity);
}
}
}
}
-------------------------------------------------------------------------------------------------------------------
-------------------------------------------------------------------------------------------------------------------
Debug custom workflow in CRM
Debug custom workflow in CRM
To debug the custom workflow in CRM on premises and online is different ways.
To debug the custom workflow in CRM on premises and online is different ways.
CRM OnPremises
In CRM on-premises sever attach the below process to debug custom workflow.
- w3wp.exe
- crmAsyncService.exe
and trigger the workflow
If the custom workflow is configured as "Sync" then you have to attach w3wp.exe process in the visual studio.
This is way can't be use in CRM online.
CRM OnLine:
With the help of "plugin registration" we can debug the custom workflow.I have mentioned below the steps to debug it.
You must first define/register your custom workflow in the CRM by adding steps in your process.
Step:1 Open the plugin registration tool and connect to CRM online. Click install profiler if it not installed already
Step:2 Once you installed profiler you can select the plugins profiler and click "profile workflow"
Step:3 In profile settings window select your workflow in the workflow lookup and steps will be automatically populate check it.
If steps is not populate then you have to use your custom workflow in the CRM and come again and do it.
Step:4 Check the persist to entity and click ok button
Step:5 After click ok button. If the error occurs
"Automatic workflow cannot be published if no activation parameters have been specified" then you have to configure workflow in CRM
as "OnDemand" to solve this error.
as "OnDemand" to solve this error.
Step:6 Goto process now you can see your selected added with name embedded with profiled.
Step:7 Thats it.Now you can trigger the workflow.
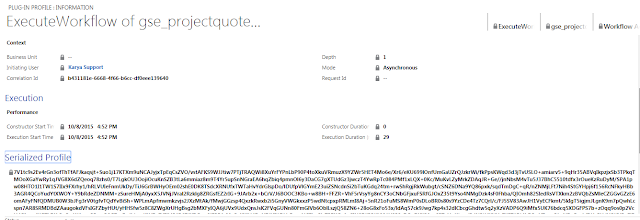
Step:8 Once you triggered the workflow. Go to "plugin profiles" and open relevant plugin record.
Step:9 Click on the “Serialized Profile” tab and copy the value specified in the “Serialized Profile”
.
Step 10. Create a new text file and copy the “Serialized Profile” value into it and then save the file.
Step 11. Go back to the Plugin Registration Tool, select the profile created earlier and click on the Debug button.
Step 12 In the opened Debug window, select the newly created text file in the Profile Location field and the Custom Workflow Activity
assembly in the Workflow Activity field.
Step 13 Open your Custom Workflow Activity project using Visual Studio.
Step 14 Put the break point in your code and attach the debugger to the Plugin Registration Tool process
and click the Start Execution button in the Plugin Registration Tool debug window.
The below steps are how to trigger the "OnDemand" workflow in the CRM
Step:1 Open any entity click "Run workflow".
Step:2 Select your custom workflow and click add now workflow get trigger.